The HTML portion of ASP.NET Web pages can contain a mix of Web controls, user controls, and HTML syntax. When an ASP.NET Web page is visited for the first time, or for the first time after a change has been made to the HTML portion of the Web page, a class is automatically created from the HTML portion. This class, which is derived from the Web page's code-behind class, constructs the Web page's control hierarchy based on the declarative syntax in the HTML portion.
For example, imagine that a Web page had the following markup in its HTML portion:
<html>
<body>
<form runat="server">
Name: <asp:TextBox runat="server" id="name"></asp:TextBox>
<br />
<asp:Button runat="server" Text="Submit"></asp:Button>
</form>
</body>
</html>
This would generate the following control hierarchy:
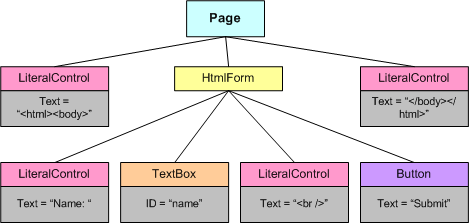
Figure 2. Controls hierarchy on page
Notice that the HTML markup is converted into LiteralControl instances, the Web Form into an HtmlForm object, and the TextBox and Button Web controls into their respective classes.
Once this control hierarchy is created, the ASP.NET Web page can be rendered by recursively invoking the RenderControl(HtmlTextWriter) method of each of the controls in the hierarchy. When a control's RenderControl(HtmlTextWriter) method is invoked, the control generates its HTML markup and appends it to the HtmlTextWriter object. Realize that each time an ASP.NET Web page is requested, the page's corresponding class is invoked causing the control hierarchy to be created and rendered.
User controls work in a similar fashion to ASP.NET Web pages. When a user control is visited for the first time, or for the first time after the HTML portion has been changed, the user control is compiled into a class that is derived from the user control's code-behind class. Like the ASP.NET Web page's autogenerated class, the user control's autogenerated class constructs a control hierarchy based on the HTML portion of the user control. In essence, a user control has its own control hierarchy.
Imagine, then, that we had a user control with the following HTML markup:
<asp:Label runat="server" id="lblCategoryName"></asp:Label>
<br />
<asp:Label runat="server" id="Description"></asp:Label>
The user control's control hierarchy, then, would look like the following:
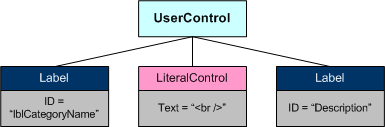
Figure 3. Control Hierarchy for user control
Recall that an ASP.NET Web page's HTML portion can contain not only Web controls and HTML syntax, but user controls as well. user controls are entered into the control hierarchy as an instance of the user control's auto generated class. So, imagine we augmented our previous ASP.NET Web page example so that after the Button Web control was an instance of the user control whose declarative syntax we just discussed. This would cause the Web page's control hierarchy to look like the following:
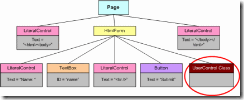
Figure 4. Updated control hierarchy for page
After the user control class is added to the control hierarchy, the class's InitializeAsUserControl(Page) method is invoked. The InitializeAsUserControl(Page) method is defined in the System.Web.UI.UserControl class, which the autogenerated user control class is derived from indirectly. (Recall that the autogenerated user control class is derived from the user control's code-behind class; this code-behind class is derived from System.Web.UI.UserControl, similar to how an ASP.NET Web page's code-behind class is derived from System.Web.UI.Page.) The InitializeAsUserControl(Page) method generates the user control's class hierarchy and assigns the user control's Page property to the passed in Page instance, thereby allowing the user control to programmatically reference the Page to which it belongs. After InitializeAsUserControl(Page) runs, the Web page's control hierarchy looks like:
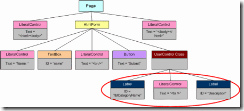
Figure 5. Updated page hierarchy after initializing the user control
Notice that once the user control is affixed to the control hierarchy and builds up its own control hierarchy, the page's control hierarchy appears just as it would if a user control hadn't been used, but instead had its HTML portion entered directly into the page's HTML portion. With this complete hierarchy, the Web page can be rendered by recursively calling the RenderControl(HtmlTextWriter) method of each control in the hierarchy.
Now that we've seen how a user control is handled underneath the covers, let's take a look at some of the more advanced concepts of user controls, starting with how to respond to events raised by Web controls in a user control.